MASTA Property Setters
In addition to the MASTA property getters outlined in the Using Python with MASTA section it is also possible to create MASTA property setters. These are scripts that can be used to directly manipulate values inside MASTA.
Creating a MASTA Property Setter
A MASTA property setter requires a getter to be created first. Once the getter has been made, it can be extended to become a setter too. The following code snippet demonstrates the syntax for accomplishing this:
from mastapy import masta_property, MeasurementType
from mastapy.system_model.part_model import RootAssembly
name = 'My Property'
measurement = MeasurementType.SHORT_LENGTH
@masta_property(name, measurement=measurement)
def my_property(root_assembly: RootAssembly) -> float:
return 0.0
@my_property.setter
def my_property(root_assembly: RootAssembly, new_value: float):
pass
The setter method must have the same name as the getter (in this instance, my_property
) and must have two parameters with the same types as the parameter and return type of the getter. Errors will be raised if these conditions are not satisfied.
Alternatively, you can generate a MASTA property setter using the same interface as the getter generator.
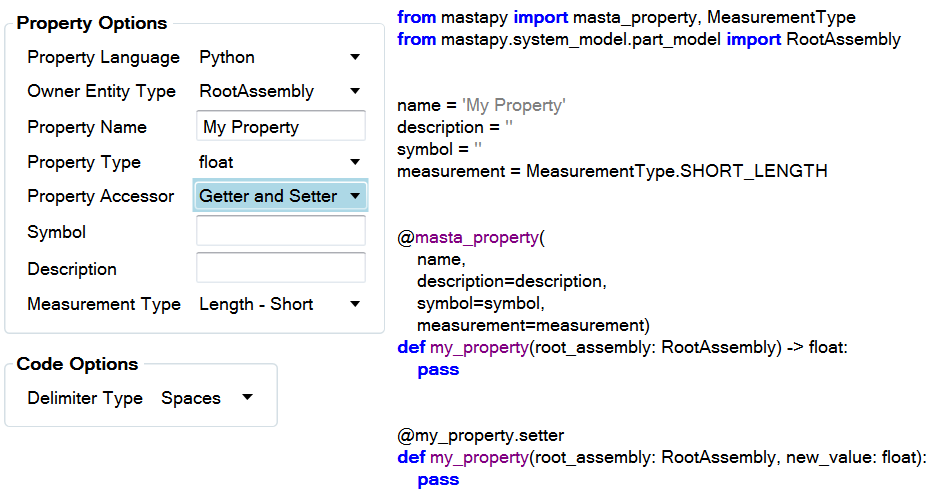
In the current version of MASTA (13.0) it is not recommended to use print
statements within your setters. Use a debugger where possible.
Using a MASTA Property Setter
MASTA property setters can only be added to a custom table in the editor tab. General information on how to do this can be found in the .NET documentation.
They can be added to a custom table using the Edit Custom Table/Chart dialog:
Select the correct category for your property.
Double-click your property from the User Defined items section.
Your property should now appear in the editor preview.
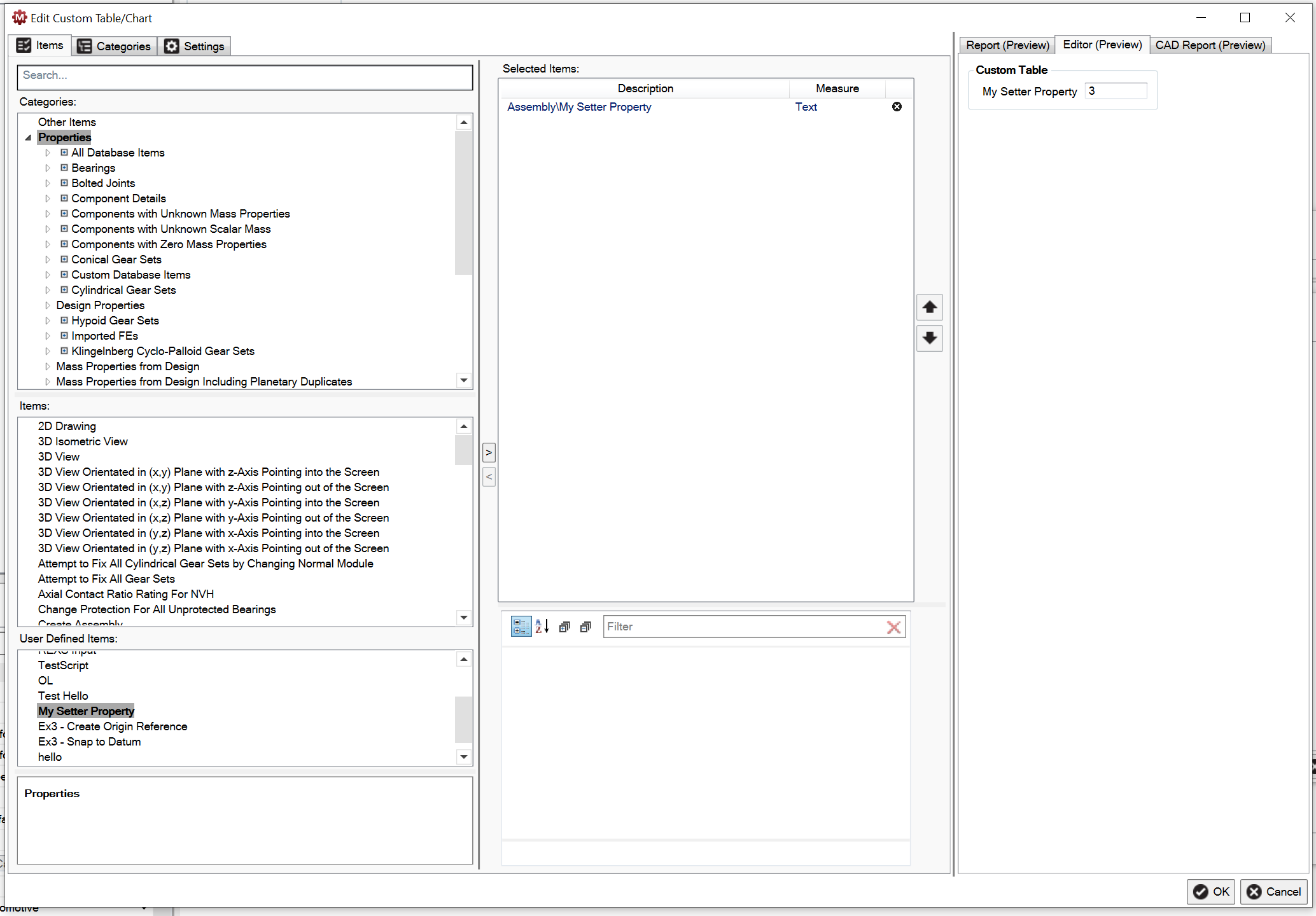
For a MASTA property setter to appear in the editor, it is important for the associated getter to return a value other than None
. Any property that returns None
will be hidden inside the editor.